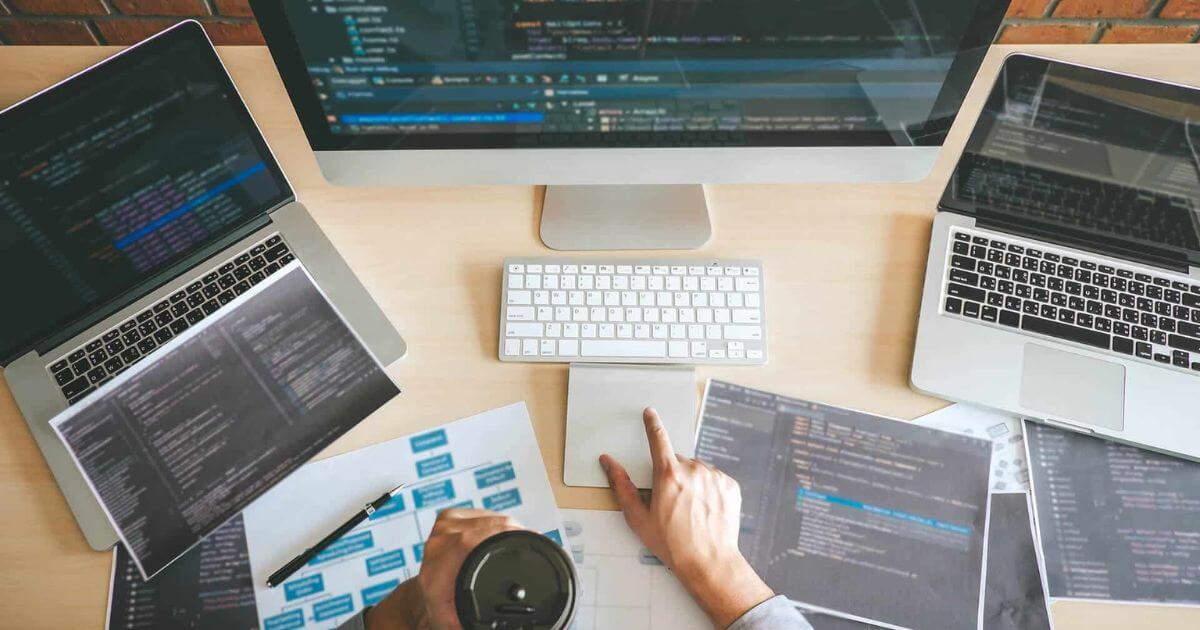
How to Read Binary Code
Understanding Binary Code for Beginners
Table of Contents
- Introduction to Binary Code
- Binary Number System Basics
- How to Convert Binary to Decimal
- Practical Examples of Binary Code
- Binary Code in Computing
- Related Tools
Introduction to Binary Code
Binary code is the foundation of computer systems. It consists of only two numbers: 0 and 1. Each digit in a binary number is called a bit. Understanding binary code is essential for anyone looking to delve into computer science, electronics, or data encoding.
Binary Number System Basics
Binary, also known as base-2, is the simplest form of number system used in digital electronics and computers. Here are the basics:
- Bits: The smallest unit in binary, representing either 0 or 1.
- Bytes: A group of 8 bits, e.g.,
11001010
.
Example of a Binary Number: 1011
- Each digit represents an increasing power of 2 from right to left.
- The rightmost bit (least significant bit) represents (2^0).
- The leftmost bit (most significant bit) represents (2^3).
Binary to Decimal Conversion Example:
- (1011{2} = 1 \times 2^3 + 0 \times 2^2 + 1 \times 2^1 + 1 \times 2^0 = 8 + 0 + 2 + 1 = 11{10})
How to Convert Binary to Decimal
Converting binary to decimal is straightforward. Here’s a step-by-step process:
- Write down the binary number: For example,
10110
. - List the powers of 2 from right to left: (2^0, 2^1, 2^2, 2^3, 2^4).
- Multiply each bit by its corresponding power of 2:
- (1 \times 2^4 = 16)
- (0 \times 2^3 = 0)
- (1 \times 2^2 = 4)
- (1 \times 2^1 = 2)
- (0 \times 2^0 = 0)
- Sum the results: (16 + 0 + 4 + 2 + 0 = 22)
Therefore, 10110
in binary equals 22
in decimal.
Code Example in Python:
def binary_to_decimal(binary_str):
decimal = 0
for digit in binary_str:
decimal = decimal * 2 + int(digit)
return decimal
binary_number = '10110'
print(f"The decimal equivalent of {binary_number} is {binary_to_decimal(binary_number)}")
Practical Examples of Binary Code
Example 1: Converting 1101
to decimal:
- (1 \times 2^3 = 8)
- (1 \times 2^2 = 4)
- (0 \times 2^1 = 0)
- (1 \times 2^0 = 1)
- Sum: (8 + 4 + 0 + 1 = 13)
Example 2: Binary addition of 1010
and 1101
:
1010
+ 1101
------
10011
Explanation:
- Start from the rightmost bit and add each column, carrying over any values as necessary.
Binary Code in Computing
Binary code is fundamental to computing. Here’s why:
- Data Storage: All digital data (text, images, videos) is stored in binary form.
- Data Processing: CPUs use binary arithmetic for calculations.
- Networking: Binary data is transmitted across networks.
Key Concepts:
- ASCII Encoding: Characters are represented by 7 or 8-bit binary numbers. For example, the ASCII code for 'A' is
01000001
. - Machine Language: The set of binary instructions that a computer's CPU executes.
Related Tools
Explore these tools to enhance your understanding and application of binary code:
Category | Tool | Link |
---|---|---|
Image Conversion | WebP to PNG | Link |
JPG to ICO | Link | |
Data Conversion | JSON to CSV | Link |
XML to JSON | Link | |
Text Utilities | Text Sorter | Link |
Text Repeater | Link |
Understanding binary code is essential for anyone involved in computing. Whether you're a beginner or looking to deepen your knowledge, mastering binary code opens up a world of possibilities in technology and data processing. Happy coding!